| |
GDS_KEY Mask Layout generation library
The development of GDS_KEY
is discontinued. The library has been completely reworked and is now available
as IPKISS.
Introduction
GDS_KEY is a python-based library for the generation of GDSII layouts,
including hierarchy.
As the library is based on
python, it provides for an easy way to use scripts to parametrize mask
layouts. For complex layouts, this is generally considered more fault-tolerant
than designing through a graphical user interface, as all coordinates and
parameters can be calculated automatically.
GDS_KEY can directly generate binary GDSII files, or a
ASCII representation in the KEY file format. The latter file format can be read
with a text editor or visually inspected using the
Boolean GDSII
viewer/editor.
Installation
- Install Python by downloading and executing the
installation package from
http://www.python.org/download/
- Download the GDS_KEY installer for your platform
The installation routine installs the GDS_KEY
package in the <Python>/Lib/site-packages
directory. Documentation (only a readme file at the moment)
and a number of examples are stored in the
<Python>/gds_key directory.
Use
After installation, the package can be used
in any python script with the import command:
from gds_key import *
A GDSII file consists of a list of named structures, which
each can contain any number of graphical elements. The elements can be
- Filled polygons (BOUNDARY) or lines with a finite width
(PATH)
- References to other structures in the file. These can
be either simple references (SREF) or the referenced structures can be
periodically repeated (Array reference or AREF).
- Text Labels
Because structures can contain references to other
structures, the GDSII file format is said to contain hierarchy. For complex mask
layouts, (e.g. with photonic crystals), the use of hierarchy is necessary to
keep the file size reasonable.
The following brief python script demonstrated the use of
the GDS_KEY library
from gds_key import * set_file_format(GDS_File_Format) #Write to GDSII set_grid(5E-9) # all dimensions are snapped to the grid (5nm)
set_unit(1E-6) # all dimensions are expressed in this unit (1um)
gds_write(header()) #first thing that should be written
str_el = el_rect (layer=0, center=(0,150),box_size=(200, 200.0)) #rectangle
str_el += el_circle(layer=1, center=(0,-150), radius=100) #circle
gds_write(make_str("boundaries", str_el)) #make a structure str_el = el_rect_path (layer=2, center=(0,150),
box_size=(200, 200), line_width=4.0) #rectange
str_el += el_circle_path(layer=3, center=(0,-150),
radius=100, line_width=4.0) #circle
gds_write(make_str("paths", str_el)) #make a structure with these elements
str_el = ( el_sref("boundaries", (0,0)) +
el_sref("paths", (300,0)) ) #simple references
gds_write(comment("Top layout structure"))
gds_write(make_str("layout", str_el))
gds_write(footer()) #close the file
This code is also avaliable in the file
<Python>/gds_key/examples/simple.py. You can execute the code from the
command prompt
python simple.py > simple.gds
The command gds_write() sends the generated GDSII
file to stdout, but by using the > operator you can send the
output to a file. The result of this file should look like this:
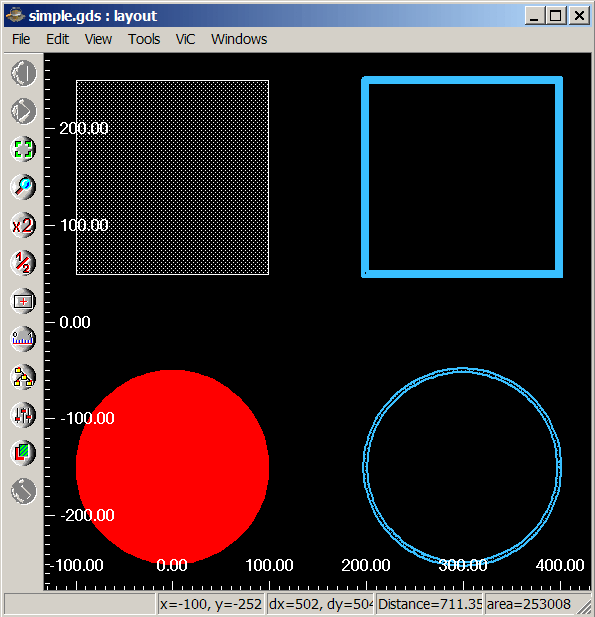
The GDS_KEY library has the following capabilities, which
are illustrated in the various example files in the <Python>/gds_key/examples
directory
- A large number of geometrical primitives, available in
functions shape_circle(...), shape_rect(...). The functions
return a Python list of coordinates. (No example file available yet)
- The shapes can be manipulated using
shape_rotate(...), shape_translate(...), ...
- el_path(...), el_boundary(...),
el_sref(...), el_aref(...), el_label(...) and el_box(...)
provide a the basic GDSII elements. (elements.py)
- Wrapper functions like el_circle(...),
el_rect_path(...) automatically generate the correct shape and feed it to
the el_boundary() and el_path() functions. (elements.py)
- GDS_KEY provides an own font which converts text to
paths. (elements.py)
- Automatic tracking of referred structures and generated
structures to validate the hierarchy of the GDSII file. A correct layout file
has only a single top level which is not referred to.
- Import of HPGL plotter files that can be converted to
paths or boundaries (hpgl.py)
- Import of existing GDSII files into a layout (import.py)
- Scalable logos of IMEC, Ghent University and the INTEC
department are built-in (logos.py).
See also
|